Implementing a Block Puzzle Solver with GWT
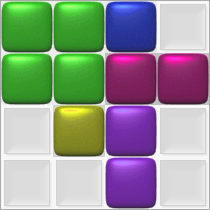
Writing a simple GUI for the Block Puzzle Solver turned out to be semi painful. I’ll go over the problems encountered while writing the puzzle solver with GWT. GWT converts Sun’s Java into browser compatible Javascript. For details of what motivated me to do this, see the writeup on the Dorito’s The Quest puzzles.
Issue #1: Browsers are slow.
Firefox 3.01 sports the fastest (or second fastest depending on who you believe) javascript interpreter around, but its still rediculously slow. Based on some simple tests it seems to be somewhere between 500-2000 times slower than Sun’s JVM. Theres really no workaround to insufficient computation power other than good algorithms.
To help with the speed problem I implemented three main features in the solver.
Block aliasing. If two unimportant identical blocks swap places, then that should not count as a new state to evaluate. Duplicate state elimination. Two states that are identical must not be re-evaluated. A-star (A) state sorting. Using A only provides a small improvement (about 10%) because block paths are always cluttered.
##Issue #2: Browsers are silly.
There are a lot of weird quirks when running code a on a browser. GWT vastly simplifies things, but there are inherent flaws it cannot fix. For one, there is no threading mechanism, this means you have to do a little bit of work then give up control and wait for a callback to do a little bit more work. If you don’t give up control fast enough then browsers terminate your javascript. This is an implementation detail and it only makes the solver slightly more complicated.
The other benefit to having threads is the obvious computational benefit, a block solver could be parallelized pretty easily.
##Issue #3: No image manipulation/creation API in browers.
In standard javascript there is no way to create or manipulate images. However, you will notice the block solver has many colorful blocks that look like chicklets. If browsers can not generate these images, then how are they being displayed?
The obvious answer is that the block images are downloaded. We generate a URL that contains hue, saturation and value of the desired block that we wish to display. The browser dynamically issues an image request for the next block color to be used. On the server the url is processed as a php script that generates the proper color block using ImageMagick.
##Issue #4: No Drag and Drop in GWT
The GWT core has no native support for drag and drop. This really perplexes me that GWT does not include any sort of drag and drop functionality. It seems to be a vital component of any interactive application.
However, there is an excellent third party library called gwt-dnd. The latest version of this library is written against the beta version of GWT. This ending up costing me a lot of time because I got stuck with an IE bug that ended up taking hours to solve.
Update: The IE bug was solved instantly after I reported it. The fix is included in release 2.5.4 (Currently a release candidate).
##Overall Assessment of GWT
I really like GWT.
- The core library is actually very well debugged and extremely usable and complete.
- It works as advertised.
- It aspires to greatness and is slowly crawling toward it.
- It’s amazing how well it works, considering javascript never works right in all browsers.
- It would work extremely well for AJAX sites.
- There are, however, the aforementioned looming problems. Of those, only issue #4 can ever really be resolved. Therefore it is currently the wrong tool for computation and fluid interactivity. A regular applet or flash would be superior for these kinds of tasks, however I’m optimistic that gwt will continue to get better.